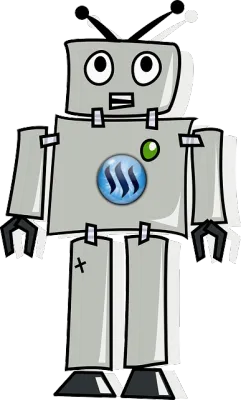
Steem requires two important elements: the account name and the password. They are crucial because all the private and public keys (owner, active, posting, memo) are derived from them. Losing any of them calls for trouble, especially the password. For instance, if we look at the key generation code in Steem-JS, we have the following function that generates the public keys:
Auth.generateKeys = function (name, password, roles) {
var pubKeys = {};
roles.forEach(function (role) {
var seed = name + role + password;
var brainKey = seed.trim().split(/[\t\n\v\f\r ]+/).join(' ');
var hashSha256 = hash.sha256(brainKey);
var bigInt = bigi.fromBuffer(hashSha256);
var toPubKey = secp256k1.G.multiply(bigInt);
var point = new Point(toPubKey.curve, toPubKey.x, toPubKey.y, toPubKey.z);
var pubBuf = point.getEncoded(toPubKey.compressed);
var checksum = hash.ripemd160(pubBuf);
var addy = Buffer.concat([pubBuf, checksum.slice(0, 4)]);
pubKeys[role] = config.get('address_prefix') + bs58.encode(addy);
});
return pubKeys;
};
And the function that generates the WIF (Wallet Import Format) private keys:
Auth.toWif = function (name, password, role) {
var seed = name + role + password;
var brainKey = seed.trim().split(/[\t\n\v\f\r ]+/).join(' ');
var hashSha256 = hash.sha256(brainKey);
var privKey = Buffer.concat([new Buffer([0x80]), hashSha256]);
var checksum = hash.sha256(privKey);
checksum = hash.sha256(checksum);
checksum = checksum.slice(0, 4);
var privWif = Buffer.concat([privKey, checksum]);
return bs58.encode(privWif);
};
Both of those functions rely on the name and password to set a seed that is hashed later to create the WIF key.
var seed = name + role + password;
Because of the one-way sha256 hash, it's extremely difficult to reverse the functions and retrieve the account name using the password, unless you have a badass supercomputer and years of patience to bruteforce.
NOTE: Since bruteforcing is unlikely, but technically possible, it's always a good practice to change your password and key set regularly, especially if you have a big account. And of course, use a very long and random password to increase the security.
So why do we need to do find the account name from the password anyway? Well, many users get approved by Steemit, they activate their account and months later, forget they even had one. Luckily, some users are smart enough to save the password, but forget what account name they created, since it's not included in the approval email, for security and privacy reasons. Then they later realize that Steemit is such a wonderful platform with much fun (and profits) that they decide to use their account at last. But oooops, no account name at hand! OMG what can they do?
Steemd.com Method
The simplest way to solve that problem is to check the history of accounts created by steem. Steemit.com uses the steem account to create all the new accounts. The entity that creates your account is your trustee. The account may have been created by a trustee other than steem, for example anonsteem, or a friend.
So, by going to https://steemd.com/@steem (or @yourtrustee), we can find all the accounts created by searching for the string create account
. What really helps is the approval email date and the assumption that the user created their account on that same day.
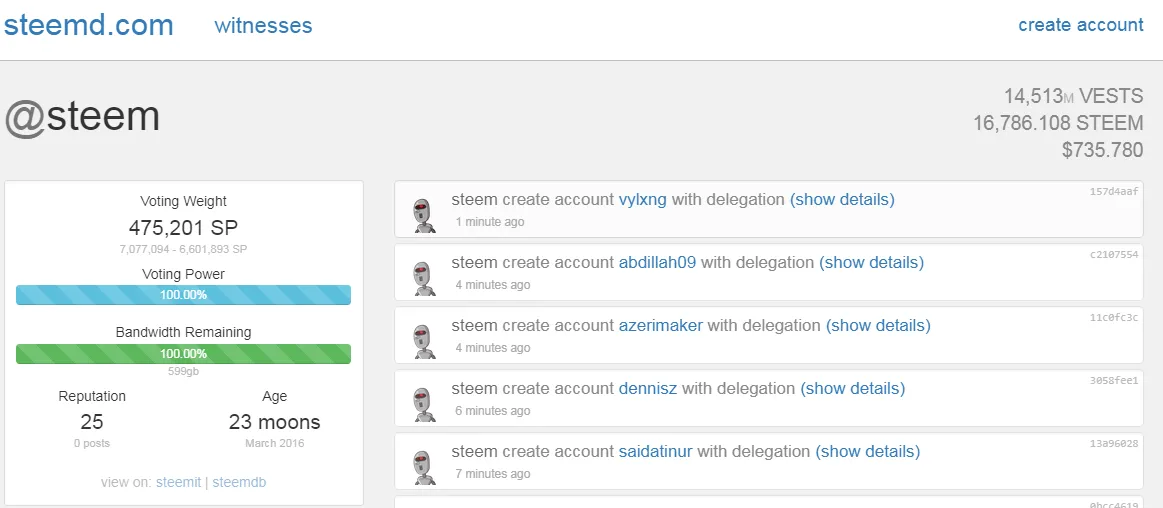
Then, we can scroll back in the steemd.com database and see if any familiar username rings a bell. The history can be found at the bottom of the page.
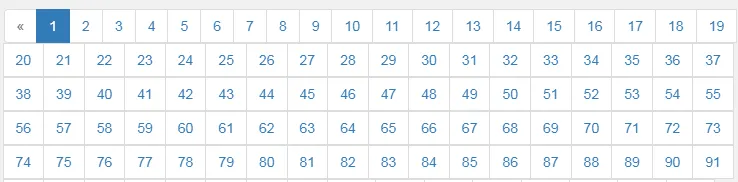
But, what if none of the accounts rings any bell? And what if the approval email was deleted? We'd have to sift through tens of thousands of accounts hoping to ring that bell, which can be daunting!
Behold the second option, the delicious part of this post. We can use some database and programming magic to find our missing account name with 100% accuracy. But how?
SteemSQL Method
We can use SteemSQL's awesome database, ran by @arcange. Please use his database responsibly, don't hammer it with crazy lengthy queries.
If we know the account creation date or range, the search can be ran with the following query:
SELECT name,created FROM Accounts WHERE created BETWEEN '2017/08/15' AND '2017/08/16' ORDER by name ASC;
That query will search all the accounts created on 2017/08/15
Alternatively, we can use this query, which gives the exact same results:
SELECT name,created FROM Accounts WHERE created BETWEEN '2017/08/15 00:00:00' AND '2017/08/15 23:59:59' ORDER by name ASC;
If you're not familiar with SQL queries, don't be scared it's not esoteric to do one. There's a nice freeware tool HeidiSQL (for Windows). Use this in the configuration and connect to the database:
Network type: Microsoft SQL Server (TCP/IP)
Hostname/IP: sql.steemit.com
User: steemit
Password: steemit
Port: 1433
Database: DBSteem
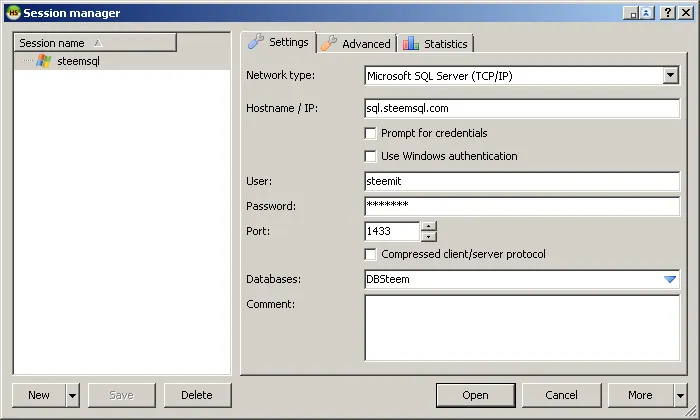
- Select the Query tab (or press Ctrl-T to open a new query tab), then paste one of the queries above (edited of course with your date range).
- Press F9 to run the query. After a few seconds, you will get a list of all accounts created (by steem or any other user) for that given date/range.
- Browse through the list and see if you can find anything that looks familiar to you.
This method is comparable to the steemd.com method, but it's blazing fast and yields sorted and concentrated results that are easier to browse. However, if none of the accounts rings any bell, what can we do? That's when we resort to SteemJS to do a more advanced search.
SteemSQL + SteemJS Method (Advanced)
This is the best method that will give us a 100% accuracy, provided that the password is the correct one. The idea is to programmatically get each account created in a date range (using SteemSQL). Then, loop through each account and its public memo key (I chose the memo key, but we can do that with any of the other public keys). While looping, we use the Auth.getPrivateKeys function from SteemJS. It's a function that derives all the private and public keys for a given name+password+role(s). We're interested in fetching the calculated public memo key, which we compare to the blockchain public memo key that we have in the SQL database. If they match, then we found the missing account name.
CODE
https://github.com/Jolly-Pirate/steem-recover-account
/*
STEEM Account Recovery From Password v1.0 - by @drakos
A NodeJS script to recover a forgotten account name using the password. The script uses steemsql.com's database.
SETUP:
- Install NodeJS (https://nodejs.org).
- Create a folder for your project and go into it.
- Copy the script into a file, e.g. recover_account.js.
- Alternatively:
git clone https://github.com/Jolly-Pirate/steem-recover-account && cd recover-account
- Install the steem and mssql packages into your project folder (don't use mssql 4.x):
npm install steem@latest [email protected]
USAGE:
Run the script from the project's folder with:
node recover_account.js YOURPASSWORD startdate enddate
The dates are in the format year/mm/dd
For example, using a single day search:
node recover_account.js YOURPASSWORD 2017/12/01 2017/12/01
Or if searching on a range of dates (takes longer of course):
node recover_account.js YOURPASSWORD 2017/12/01 2017/12/05
*/
var steem = require("steem");
var sql = require('mssql');
steem.api.setOptions({url: "https://api.steemit.com"});
// Get arguments from command line
var args = process.argv.slice(2);
console.log(JSON.stringify(args));
var password = args[0];
var dateStart = args[1];
var dateEnd = args[2];
var benchmarkStart = new Date(); // Start benchmark
var benchmarkEnd, benchmarkDiff, created;
// Using the database from steemsql.com
var config = {
user: "steemit",
password: "steemit",
server: "sql.steemsql.com",
port: 1433,
database: 'DBSteem'
};
getAccountFromPassword(password, dateStart, dateEnd); // Run the function
function getAccountFromPassword(password, dateStart, dateEnd) {
var dbConn = new sql.Connection(config);
dbConn.connect().then(function () {
var request = new sql.Request(dbConn);
dateStart = "'" + dateStart + " 00:00:00'";
dateEnd = "'" + dateEnd + " 23:59:59'";
var query = "SELECT name,created,memo_key FROM Accounts WHERE created BETWEEN " + dateStart + " AND " + dateEnd + " ORDER BY name ASC";
request.query(query).then(function (recordSet) {
// Loop through the accounts
for (i = 0; i < recordSet.length; ++i) {
// Values on the blockchain
name = recordSet[i].name;
memokeyBlockchain = recordSet[i].memo_key;
// We only need one of the public keys, let's pick the memo key
roles = ["memo"];
memokeyDerived = steem.auth.getPrivateKeys(name, password, roles).memoPubkey;
console.log("Checking", (i + 1) + "/" + recordSet.length, name);
if (memokeyBlockchain === memokeyDerived) {
benchmarkEnd = new Date(); // End benchmark
benchmarkDiff = (benchmarkEnd - benchmarkStart) / 1000;
created = recordSet[i].created;
console.log("Found account :\t", name);
console.log("Creation date :\t", created);
console.log("Memo pubkey :\t", memokeyBlockchain);
console.log("Process took :\t", benchmarkDiff, "sec");
break; // Break the loop when the result is found
}
}
dbConn.close();
}).catch(function (err) {
console.log(err);
dbConn.close();
});
}).catch(function (err) {
console.log(err);
});
}
Usage Example
My account was approved on 2017/06/13 (yes, I keep my old important emails) and I created it on that same day because I was very eager to get started. I run the following command (notice that I use the same date for the starting and ending date range):
node recover_account.js YOURPASSWORD 2017/06/13 2017/06/13
For me, the search takes 32 seconds.
If I try a monthly search:
node recover_account.js YOURPASSWORD 2017/06/01 2017/06/30
It takes 370 seconds to find my account. Not too shabby :)
If none of the solutions work, then the password may be wrong. In that case, a new account will need to be created. That's beyond the scope of this post.